User Manual#
Turbo Turtle is a wrapper around common modeling operations in Abaqus [1] and Cubit [2]. As much as possible, the work for each subcommand is perfomed in Python 3 [3] to minimize solution approach duplication. Turbo Turtle makes a best effort to maintain common behaviors and features across each third-party software’s modeling concepts.
Quick Start#
View the CLI usage
$ turbo-turtle -h $ turbo-turtle docs -h $ turbo-turtle geometry -h $ turbo-turtle cylinder -h $ turbo-turtle sphere -h $ turbo-turtle partition -h $ turbo-turtle mesh -h $ turbo-turtle image -h $ turbo-turtle merge -h $ turbo-turtle export -h
Installation#
This project builds and deploys as a Conda package [4, 5].
Turbo-Turtle can be installed in a Conda environment with the Conda package manager. See the Conda installation and Conda environment management documentation for more details about using Conda.
$ conda install --channel conda-forge turbo_turtle
Examples: Abaqus#
Turbo Turtle was originally written using Abaqus as the backend modeling and meshing software. Most of the interface options and descriptions use Abaqus modeling concepts and language. See the Command Line Utilities documentation for additional subcommands and options.
Three-dimensional sphere#
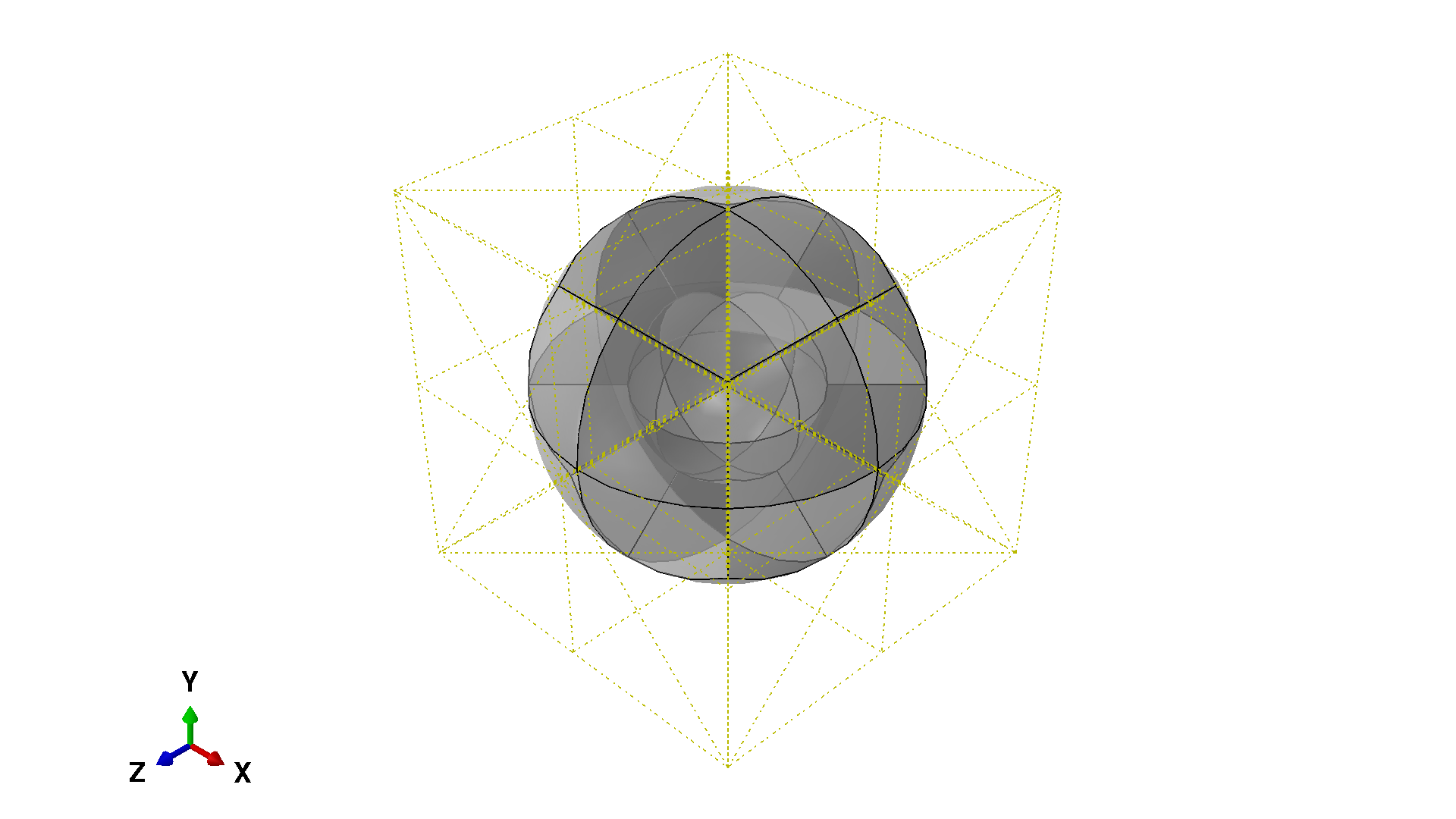
Create the geometry
turbo-turtle sphere --inner-radius 1 --outer-radius 2 --output-file sphere.cae --model-name sphere --part-name sphere
Partition the geometry
turbo-turtle partition --input-file sphere.cae --output-file sphere.cae --model-name sphere --part-name sphere
Mesh the geometry
turbo-turtle mesh --input-file sphere.cae --output-file sphere.cae --model-name sphere --part-name sphere --element-type C3D8 --global-seed 0.15
Create an assembly image
turbo-turtle image --input-file sphere.cae --output-file sphere.png --model-name sphere --part-name sphere
Export an orphan mesh
turbo-turtle export --input-file sphere.cae --model-name sphere --part-name sphere
Two-dimensional, axisymmetric sphere#
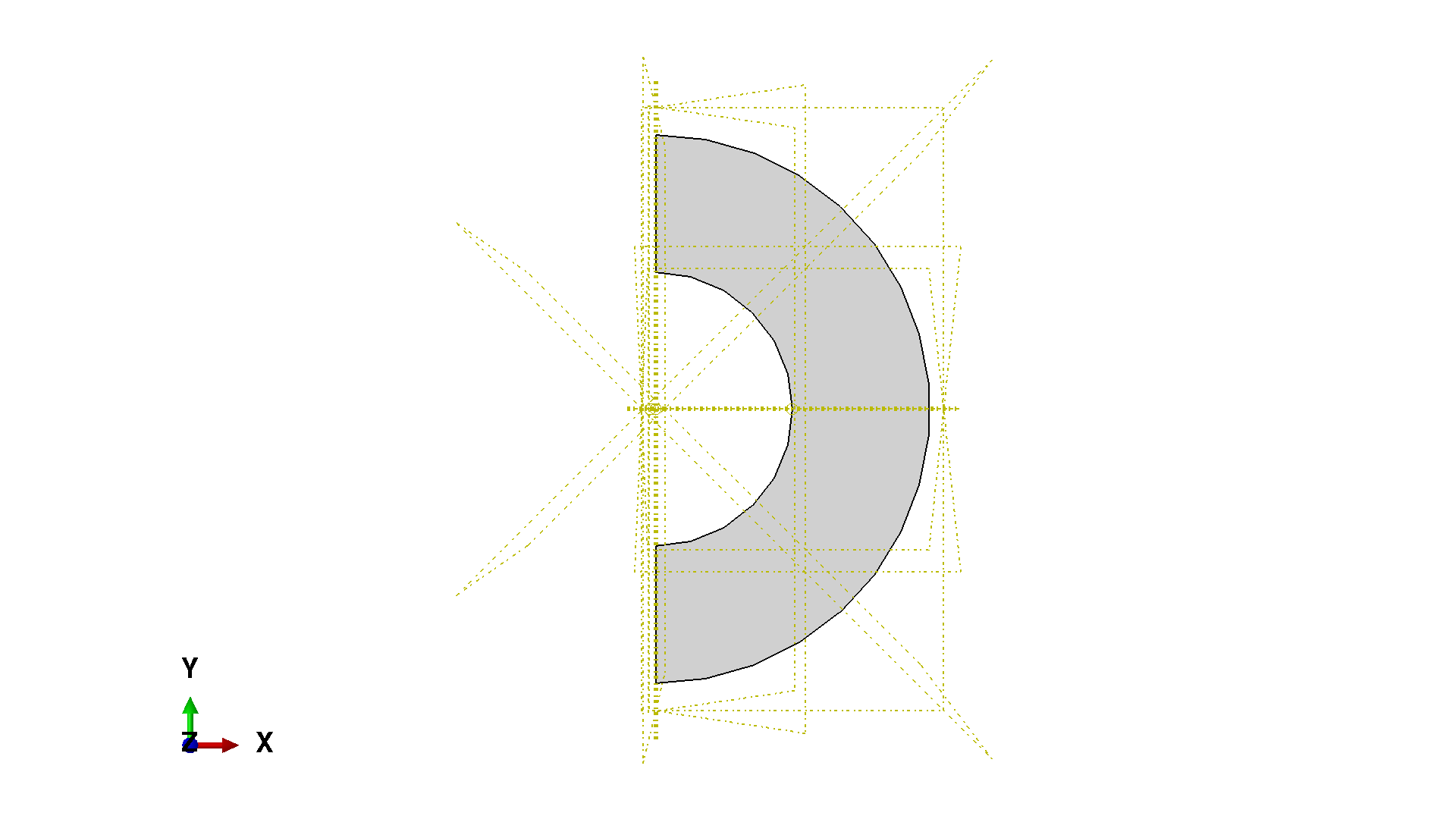
Create the geometry
turbo-turtle sphere --inner-radius 1 --outer-radius 2 --output-file axisymmetric.cae --model-name axisymmetric --part-name axisymmetric --revolution-angle 0
Partition the geometry
turbo-turtle partition --input-file axisymmetric.cae --output-file axisymmetric.cae --model-name axisymmetric --part-name axisymmetric
Mesh the geometry
turbo-turtle mesh --input-file axisymmetric.cae --output-file axisymmetric.cae --model-name axisymmetric --part-name axisymmetric --element-type CAX4 --global-seed 0.15
Create an assembly image
turbo-turtle image --input-file axisymmetric.cae --output-file axisymmetric.png --model-name axisymmetric --part-name axisymmetric
Export an orphan mesh
turbo-turtle export --input-file axisymmetric.cae --model-name axisymmetric --part-name axisymmetric
Examples: Cubit#
These examples are (nearly) identical to the Abaqus examples above, but appended with the --backend cubit
option.
Because the commands are (nearly) identical, they will be included as a single command block. See the
Command Line Utilities documentation for caveats in behavior for the Cubit implementation and translation of Abaqus
language to Cubit language. The list of commands will be expanded as they are implemented.
Note
The
--model-name
option has no corresponding Cubit concept and is ignored in all Cubit implementations.The mesh subcommand
--element-type
option maps to the Cubit meshing scheme concept. It is only used if a non-default scheme is passed: trimesh or tetmesh. Because the Abaqus and Cubit implementations share a command line parser, and the Abaqus implementation requires this option, it is always required in the Cubit implementation as well.The image subcommand must launch a Cubit window and the Cubit commands only work in APREPRO journal files, so an
output_file
.jou file is created.
Three-dimensional sphere#
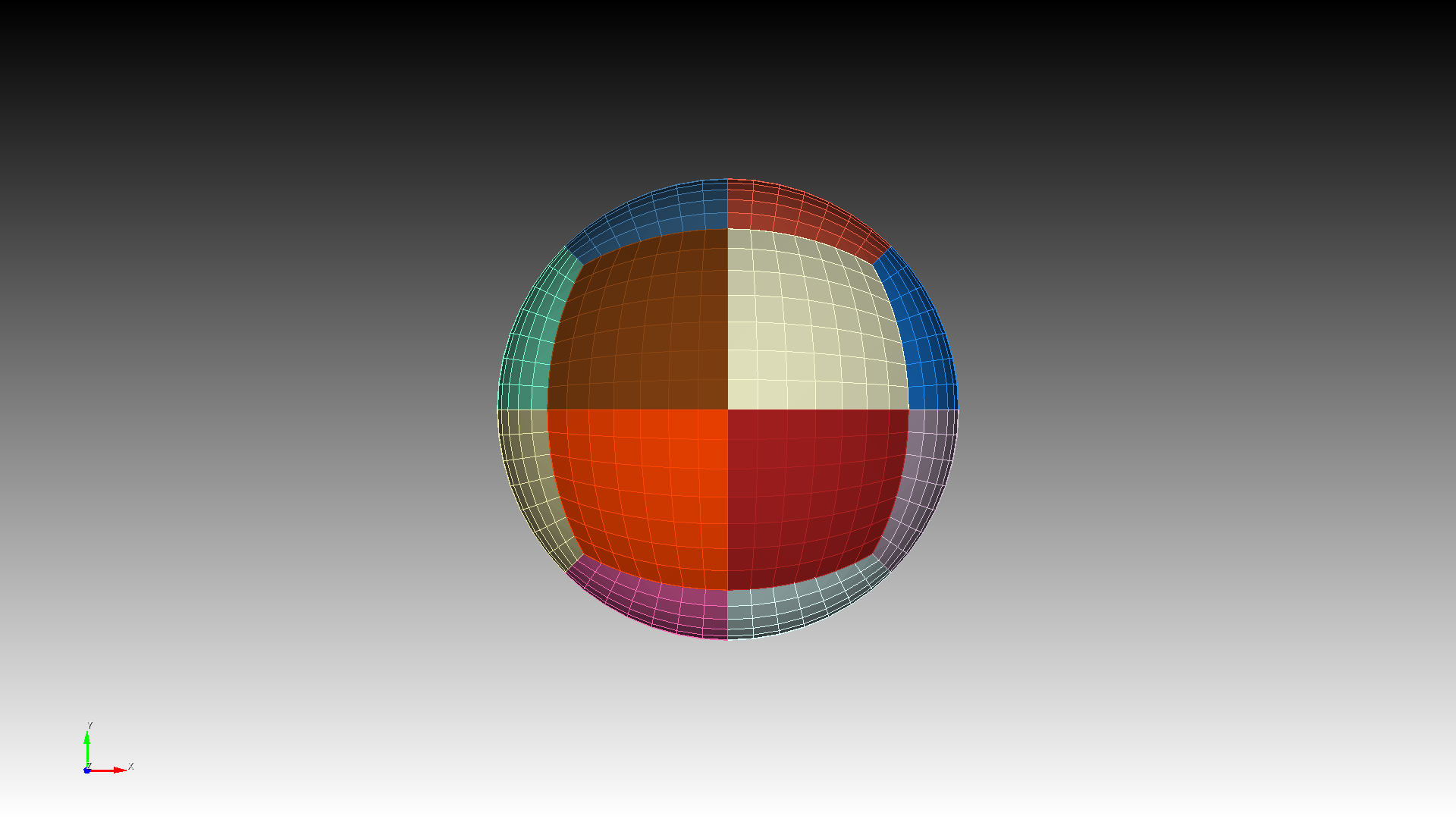
turbo-turtle sphere --inner-radius 1 --outer-radius 2 --output-file sphere.cub --part-name sphere --backend cubit
turbo-turtle partition --input-file sphere.cub --output-file sphere.cub --part-name sphere --backend cubit
turbo-turtle mesh --input-file sphere.cub --output-file sphere.cub --part-name sphere --element-type dummy --global-seed 0.15 --backend cubit
turbo-turtle image --input-file sphere.cub --output-file sphere.png --backend cubit
turbo-turtle export --input-file sphere.cub --part-name sphere --backend cubit
Two-dimensional, axisymmetric sphere#
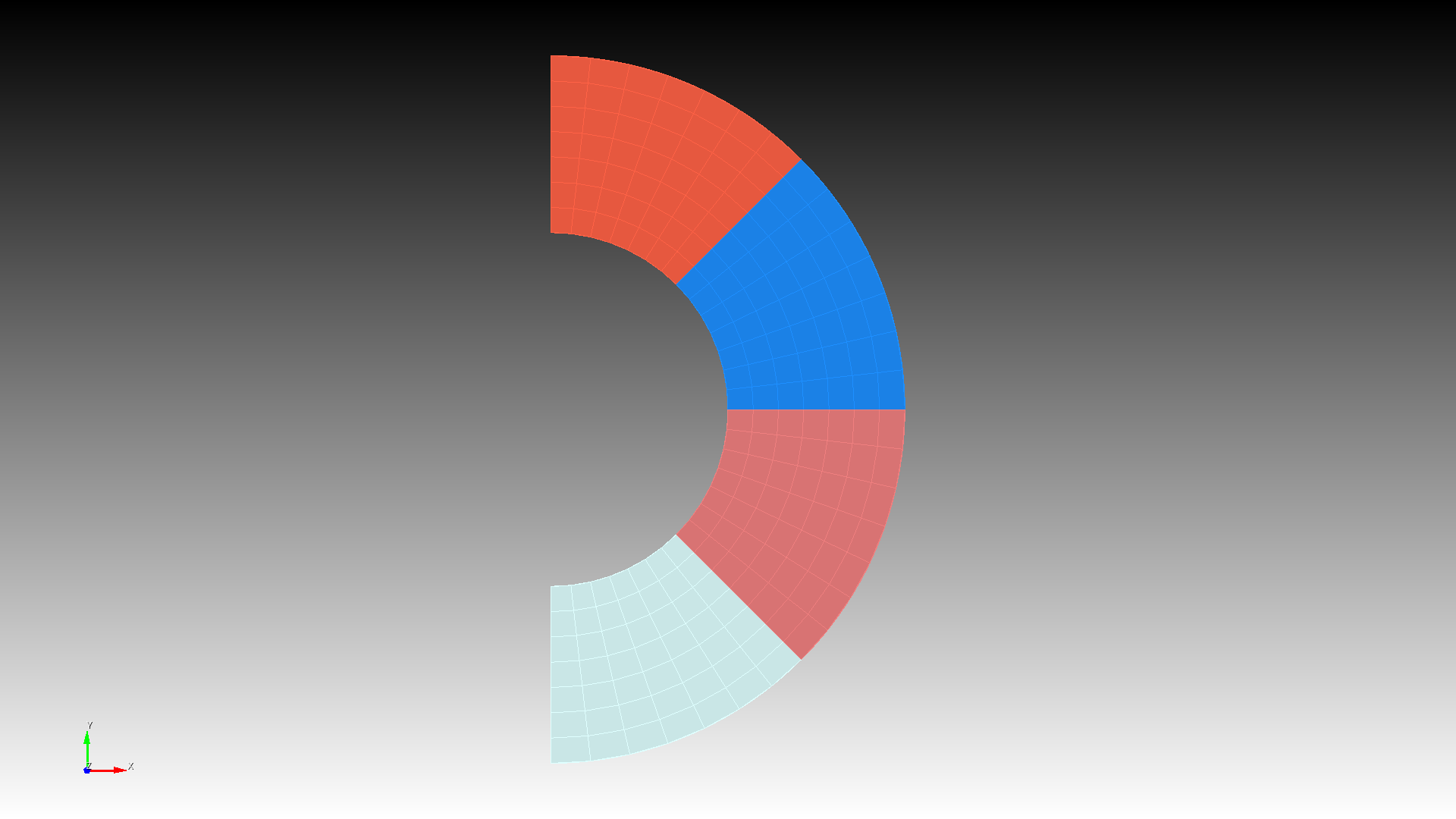
turbo-turtle sphere --inner-radius 1 --outer-radius 2 --output-file axisymmetric.cub --part-name axisymmetric --revolution-angle 0 --backend cubit
turbo-turtle partition --input-file axisymmetric.cub --output-file axisymmetric.cub --part-name axisymmetric
turbo-turtle mesh --input-file axisymmetric.cub --output-file axisymmetric.cub --part-name axisymmetric --element-type dummy --global-seed 0.15
turbo-turtle image --input-file axisymmetric.cub --output-file axisymmetric.png --backend cubit
turbo-turtle export --input-file axisymmetric.cub --part-name axisymmetric
SCons extensions#
Turbo Turtle includes extensions to the SCons [6, 7] build system in the External API scons_extensions module. These may be used when importing Turbo Turtle as a Python package in an SCons configuration file. For example:
SConstruct
1#! /usr/bin/env python
2import os
3import inspect
4import pathlib
5
6import waves
7import turbo_turtle
8
9
10# Accept command line options with fall back default values
11AddOption(
12 "--build-dir",
13 dest="variant_dir_base",
14 default="build",
15 nargs=1,
16 type=str,
17 action="store",
18 metavar="DIR",
19 help="SCons build (variant) root directory. Relative or absolute path. (default: '%default')"
20)
21AddOption(
22 "--turbo-turtle-command",
23 dest="turbo_turtle_command",
24 default="turbo-turtle",
25 nargs=1,
26 type="string",
27 action="store",
28 metavar="DIR",
29 help="Override for the Turbo-Turtle command (default: '%default')"
30)
31
32env = Environment(
33 ENV=os.environ.copy(),
34 variant_dir_base=pathlib.Path(GetOption("variant_dir_base"))
35 turbo_turtle_command=GetOption("turbo_turtle_command"),
36)
37
38env["abaqus"] = waves.scons_extensions.add_program(env, ["/apps/abaqus/Commands/abq2024", "abq2024"])
39env["cubit"] = waves.scons_extensions.add_cubit(env, ["/apps/Cubit-16.16/cubit", "cubit"])
40
41env.Append(BUILDERS={
42 "TurboTurtleSphere": turbo_turtle.scons_extensions.sphere(
43 program=env["turbo_turtle_command"],
44 options="--model-name ${model_name} --part-name ${part_name} --backend ${backend}",
45 abaqus_command=[env['abaqus']],
46 cubit_command=[env['cubit']],
47 ),
48 "TurboTurtlePartition": turbo_turtle.scons_extensions.partition(
49 program=env["turbo_turtle_command"],
50 options="--model-name ${model_name} --part-name ${part_name} --backend ${backend}",
51 abaqus_command=[env['abaqus']],
52 cubit_command=[env['cubit']],
53 ),
54 "TurboTurtleMesh": turbo_turtle.scons_extensions.mesh(
55 program=env["turbo_turtle_command"],
56 options="--model-name ${model_name} --part-name ${part_name} --backend ${backend} --global-seed ${global_seed}",
57 abaqus_command=[env['abaqus']],
58 cubit_command=[env['cubit']],
59 ),
60 "TurboTurtleImage": turbo_turtle.scons_extensions.image(
61 program=env["turbo_turtle_command"],
62 options="--model-name ${model_name} --part-name ${part_name} --backend ${backend}",
63 abaqus_command=[env['abaqus']],
64 cubit_command=[env['cubit']],
65 ),
66 "TurboTurtleExport": turbo_turtle.scons_extensions.export(
67 program=env["turbo_turtle_command"],
68 options="--model-name ${model_name} --part-name ${part_name} --backend ${backend}",
69 abaqus_command=[env['abaqus']],
70 cubit_command=[env['cubit']],
71 )
72})
73
74for backend, extension in [("abaqus", "cae"), ("cubit", "cub")]:
75 build_dir = env["variant_dir_base"] / backend
76 SConscript("SConscript", variant_dir=build_dir, exports=["env", "backend", "extension"], duplicate=False)
77
78env.Default()
79
80waves.scons_extensions.project_help()
SConscript
1#! /usr/bin/env python
2Import("env", "backend", "extension")
3
4workflow = []
5
6workflow.extend(env.TurboTurtleSphere(
7 target=[f"sphere_geometry.{extension}"],
8 source=["SConscript"],
9 inner_radius=1.,
10 outer_radius=2.,
11 model_name="sphere",
12 part_name="sphere",
13 backend=backend
14))
15
16workflow.extend(env.TurboTurtlePartition(
17 target=[f"sphere_partition.{extension}"],
18 source=[f"sphere_geometry.{extension}"],
19 model_name="sphere",
20 part_name="sphere",
21 backend=backend
22))
23
24workflow.extend(env.TurboTurtleMesh(
25 target=[f"sphere_mesh.{extension}"],
26 source=[f"sphere_partition.{extension}"],
27 element_type="C3D8",
28 global_seed=0.15,
29 model_name="sphere",
30 part_name="sphere",
31 backend=backend
32))
33
34workflow.extend(env.TurboTurtleImage(
35 target=[f"sphere_mesh.png"],
36 source=[f"sphere_mesh.{extension}"],
37 model_name="sphere",
38 part_name="sphere",
39 backend=backend
40))
41
42workflow.extend(env.TurboTurtleExport(
43 target=[f"sphere.inp"],
44 source=[f"sphere_mesh.{extension}"],
45 model_name="sphere",
46 part_name="sphere",
47 backend=backend
48))
49
50env.Alias(backend, workflow)